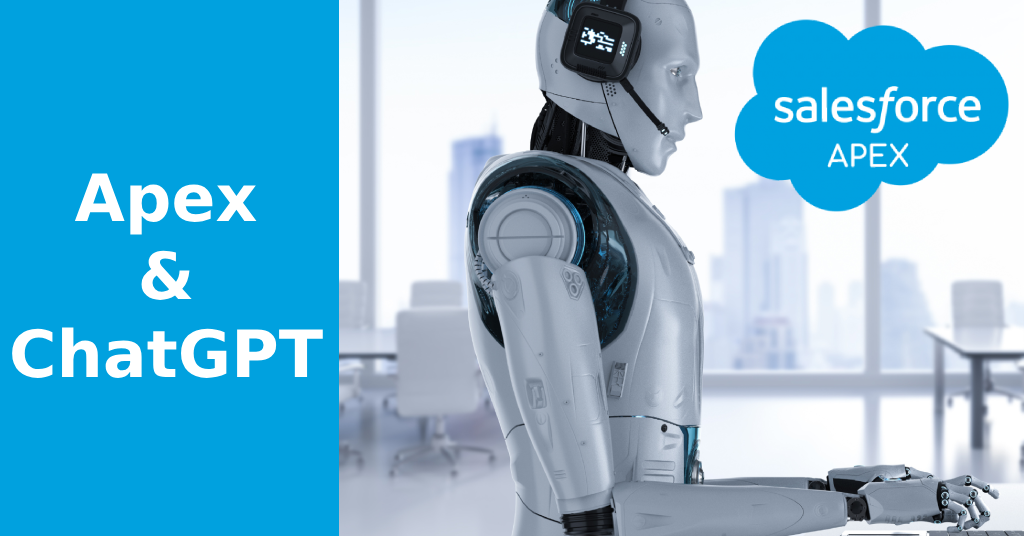
Learning to code in Salesforce Apex can feel like drinking from a firehose. But with ChatGPT to write Salesforce Apex code, things get way easier—especially for beginners. This guide walks you through exactly how I use ChatGPT to write and improve Apex code, with real prompts, examples, and key considerations.
If you’re new to Salesforce development, this is your shortcut to building confidence with the help of AI.
📘 What is Salesforce Apex?
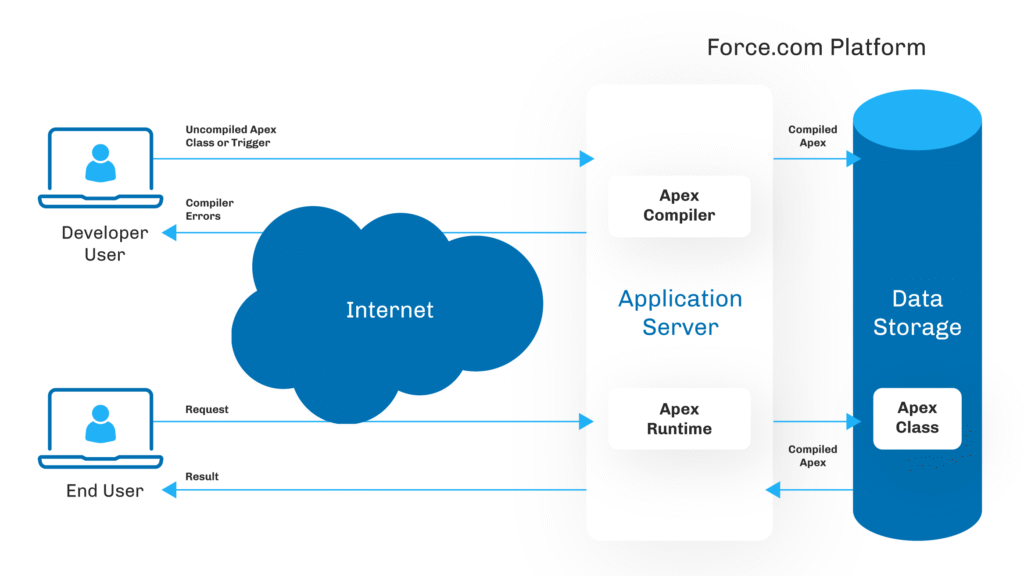
Salesforce Apex is a strongly typed, object-oriented language used to build custom functionality on the Salesforce Platform. It’s like Java, but built specifically for CRM logic and automation.
You’ll use Apex to:
- Build triggers on objects
- Create custom business logic
- Integrate with external systems via APIs
If you’re still setting up, check out this free Salesforce Developer Org Setup Guide to get started.
⚡ Why Write Apex Code Using ChatGPT?
Using ChatGPT to write Salesforce Apex code has completely changed how I approach development.
Here’s why:
- ⚡ Speed: Get boilerplate or complex logic in seconds
- 🧠 Learning: Ask “why” or “how” to understand what’s happening
- 🔧 Refactor or improve old code
- 📑 Write test classes, documentation, and comments
Instead of Googling syntax or copying from StackOverflow, I now start my logic inside ChatGPT.
🧰 What You’ll Need
Before we start prompting:
- ✅ Free ChatGPT Account
- ✅ Salesforce Developer Org
- ✅ Optional: VS Code + Salesforce Extensions
💬 Best Prompts for Using ChatGPT to Write Salesforce Apex Code
Here are some plug-and-play prompts you can use to get Apex code generated fast.
🔹 Prompt: Create a Simple Apex Class
Prompt to use:
“Write a Salesforce Apex class to assign leads to users based on lead source.”
ChatGPT might return:
public class LeadAssigner {
public static void assignLeads(List<Lead> leads) {
for (Lead l : leads) {
if (l.LeadSource == 'Web') {
l.OwnerId = '005XXXXXXXXXXXX'; // Web team
} else {
l.OwnerId = '005YYYYYYYYYYYY'; // Default team
}
}
}
}
🔍 Pro Tip: Ask follow-up prompts like:
“Can you bulkify this class for governor limits?”
🔹 Prompt: Create a Trigger
Prompt:
“Write an Apex trigger before inserting contacts that ensures email field is not blank.”
Response Example:
apexCopyEdittrigger ValidateContactEmail on Contact (before insert) {
for (Contact c : Trigger.new) {
if (String.isBlank(c.Email)) {
c.addError('Email is required.');
}
}
}
🔹 Prompt: Generate a Test Class
Prompt:
“Write a test class for the LeadAssigner Apex class with 75%+ coverage.”
Sample Result:
apexCopyEdit@isTest
public class LeadAssignerTest {
@isTest
static void testAssignLeads() {
Lead l = new Lead(LastName='Test', Company='OpenAI', LeadSource='Web');
insert l;
List<Lead> leads = [SELECT Id, LeadSource FROM Lead];
LeadAssigner.assignLeads(leads);
// Add asserts as needed
}
}
💡 Follow-up idea:
“Add negative test cases and assert owner assignment.”
🔄 Real Example: Using ChatGPT to Write Salesforce Apex Code for Triggers
Here’s a full-use case I generated with ChatGPT to write Salesforce Apex code that prevents deleting Accounts with active Opportunities.
- Prompt:
“Create an Apex trigger on Account that prevents deletion if the account has open opportunities.”
- ChatGPT Output:
apexCopyEdittrigger PreventAccountDelete on Account (before delete) {
Set<Id> accountIds = new Set<Id>();
for (Account acc : Trigger.old) {
accountIds.add(acc.Id);
}
Map<Id, Opportunity> opps = new Map<Id, Opportunity>(
[SELECT Id, AccountId FROM Opportunity WHERE AccountId IN :accountIds AND StageName != 'Closed Won']
);
for (Account acc : Trigger.old) {
if (opps.containsKey(acc.Id)) {
acc.addError('This account has open opportunities and cannot be deleted.');
}
}
}
🔥 Now paste that into your Dev org and test!
⚠️ Pros, Cons & Things to Watch Out For with Salesforce Apex prompts
✅ Pros
- Saves time on boilerplate code
- Great for quick drafts or refactoring ideas
- Excellent learning tool (ask “what does this code do?”)
- Can generate documentation and comments
⚠️ Cons
- Doesn’t fully understand Salesforce governor limits
- Sometimes suggests deprecated or insecure methods
- Doesn’t connect to your Salesforce org — no real-time validation
Golden rule: Always review and test everything in your org.
🧭 Best Practices for Using ChatGPT to Write Salesforce Apex Code
- Always validate output in a Dev org. Don’t trust blindly.
- Ask for multiple versions. E.g., “Can you optimize that with maps?”
- Use it for learning. Ask why it chose a certain method or syntax.
- Avoid copy-pasting into production without test coverage and peer review.
✅ Final Thoughts on using ChatGPT to Write Salesforce Apex code
If you’re just starting out with Salesforce Apex, ChatGPT can be a game-changer. It’s not a replacement for understanding Apex, but it’s a fantastic tool to help you write, learn, and experiment faster.
Pairing your beginner mindset with ChatGPT’s speed means you’ll write better Apex sooner—and with less frustration.
If you are ready to learn more about salesforce checkout my other articles here.
💬 ChatGPT Apex Code FAQs
❓Can ChatGPT write Salesforce Apex code accurately?
Yes, ChatGPT can write functional Apex code for many common use cases. However, it’s not always perfect—especially with Salesforce-specific nuances like governor limits. Always validate and test generated code in a Developer Org.
❓ Is it safe to use ChatGPT-generated Apex code in production?
No. ChatGPT is great for drafts and inspiration, but its code should never go straight into production without manual review, testing, and security validation.
❓How do I prompt ChatGPT to write better Apex code?
Be specific in your prompts. Include object names, desired logic, trigger contexts, and expected outcomes. For example: “Write a before insert trigger on Contact that validates the Email field.”
❓Does ChatGPT understand Salesforce governor limits?
Not completely. It sometimes generates non-bulkified code or code that risks hitting limits. Prompt ChatGPT to “bulkify” logic or ask about governor-safe alternatives.
❓Can I use ChatGPT to write test classes for Salesforce Apex?
Absolutely. ChatGPT can generate basic test classes with test data and coverage logic. You may need to fine-tune them to meet specific coverage and data setup needs for your org.